이전 포스팅에서는 GPIO에 LED와 Switch를 연결하여 실습을 진행하였습니다.
이번 실습은 Buzzer와 Photo resistor, Ultrasonic sensor를 이용한 실습을 진행해보겠습니다.
Buzzer
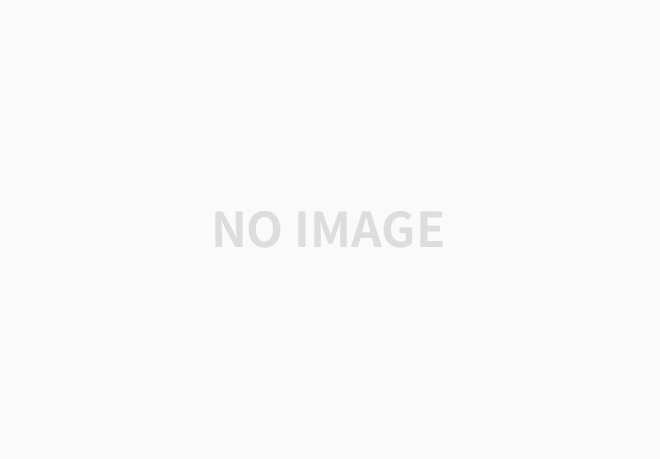
- 작은 소리를 발생하는 간단한 구조의 소자
- Active type: 회로가 내장되어 있어 전원만 연결하면 소리 출력
- Passive type: 입력 주파수에 따라 다양한 tone 의 소리를 출력
Buzzer 실습
Piezo Buzzer를 이용해 <학교종이 땡땡땡> 노래를 출력하는 실습을 진행해보도록 하겠습니다.
실습에 필요한 회로도 입니다.
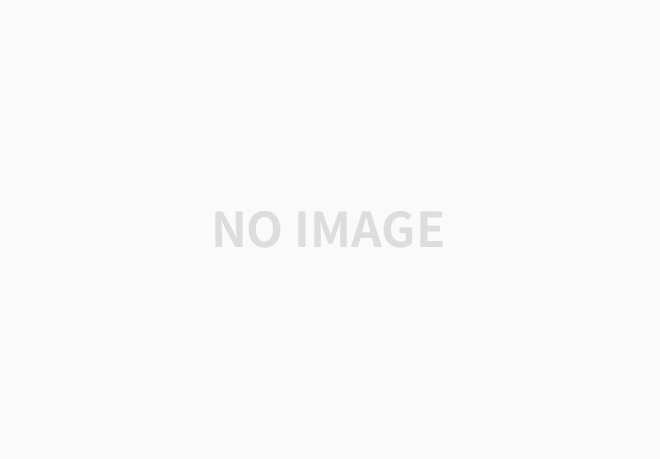
wiringmusic.c이라는 c파일을 만들어 코드를 작성해보겠습니다.
#include <wiringPi.h>
#include <softTone.h>
#define SPKR 6 /* GPIO25 */
#define TOTAL 32 /* 학교종의 전체 계이름의 수 */
int notes[] =
{ /* 학교종을 연주하기 위한 계이름 */
391, 391, 440, 440, 391, 391, 329.63, 329.63, \
391, 391, 329.63, 329.63, 293.66, 293.66, 293.66, 0, \
391, 391, 440, 440, 391, 391, 329.63, 329.63, \
391, 329.63, 293.66, 329.63, 261.63, 261.63, 261.63, 0
};
int musicPlay( )
{
int i;
softToneCreate(SPKR); /* 톤 출력을 위한 GPIO 설정 */
for (i = 0; i < TOTAL; ++i)
{
softToneWrite(SPKR, notes[i]); /* 톤 출력 : 학교종 연주 */
delay(280); /* 음의 전체 길이만큼 출력되도록 대기 */
}
return 0;
}
int main( )
{
wiringPiSetup( );
musicPlay( ); /* 음악 연주를 위한 함수 호출 */
return 0;
}
코드를 작성하였다면, gcc를 이용해 컴파일하고 실행시켜보겠습니다.
pi@raspberrypi:~ $ gcc -o wiringmusic wiringmusic.c -lwiringPi
pi@raspberrypi:~ $ sudo ./wiringmusic
위의 명령어를 통해 프로그램을 실행시켜 음악을 연주 할 수 있습니다.
Photo resistor
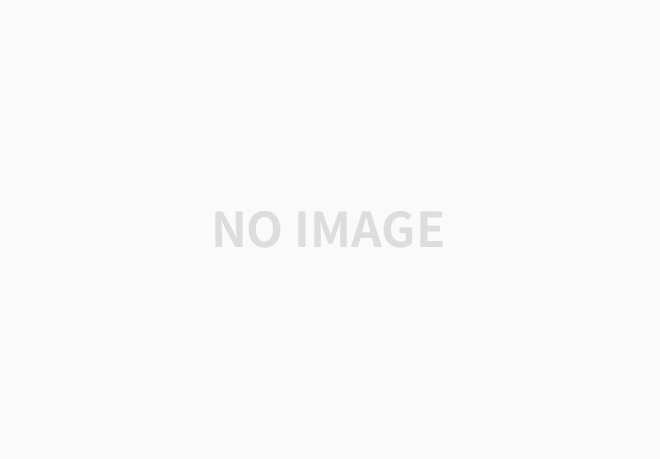
- 조도 센서 (illuminance sensor)
- 황화카드뮴 (Cds) 활용
- 빛이 없으면 저항 증가, 빛에 닿으면 저항 감소
Photo resistor 실습
Photo resistor를 이용해 빛이 감지되면 LED를 켜고, 빛이 감지되지 않는다면 LED를 끄는 실습을 진행해보겠습니다.
실습에 필요한 회로도 입니다.
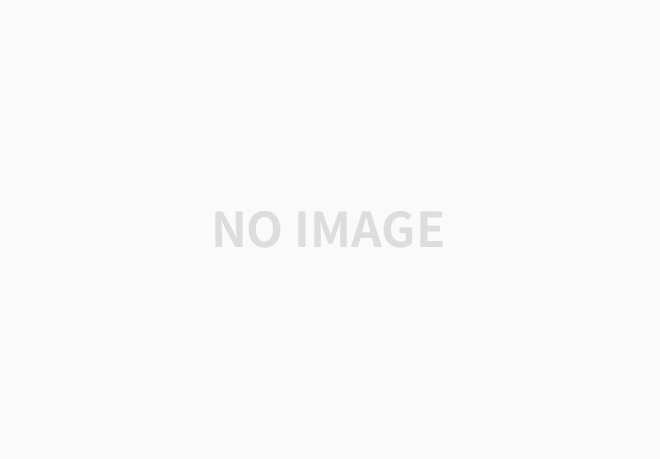
wiringlight.c이라는 c파일을 만들어 코드를 작성해보겠습니다.
#include <wiringPi.h>
#include <stdio.h>
#define SW 5 /* GPIO24 */
#define CDS 0 /* GPIO17 */
#define LED 1 /* GPIO18 */
int cdsControl( )
{
int i;
pinMode(SW, INPUT); /* Pin 모드를 입력으로 설정 */
pinMode(CDS, INPUT); /* Pin 모드를 입력으로 설정 */
pinMode(LED, OUTPUT); /* Pin 모드를 출력으로 설정 */
for (;;)
{ /* 조도 센서 검사를 위해 무한 루프를 실행한다. */
if(digitalRead(CDS) == HIGH)
{ /* 빛이 감지되면(HIGH) */
digitalWrite(LED, HIGH); /* LED 켜기(On) */
delay(1000);
digitalWrite(LED, LOW); /* LED 끄기(Off) */
}
}
return 0;
}
int main( )
{
wiringPiSetup( );
cdsControl( ); /* 조도 센서 사용을 위한 함수 호출 */
return 0;
}
코드를 작성하였다면 gcc를 이용해 컴파일하고 실행시켜보겠습니다.
pi@raspberrypi:~ $ gcc -o wiringlight wiringlight.c -lwiringPi
pi@raspberrypi:~ $ sudo ./wiringlight
위의 명령어를 통해 프로그램을 실행 시킬 수 있으며, Ctrl + C 입력하여 프로그램을 종료 할 수 있습니다.
Ultrasonic sensor
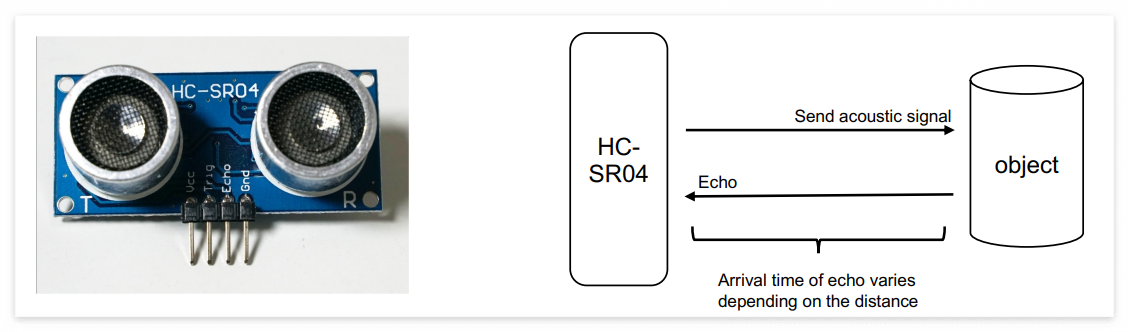
- 초음파 신호를 사용해 거리 측정하는 센서
- 가청 주파수 (20Hz ~ 20Khz) 외의 주파수 대역을 활용 (초음파)
- 특정 패턴의 초음파를 송신하고, 물체에 의해 반사되는 음파를 수신함.
- 송신 <> 수신의 시간차와 음파의 전달 속도를 바탕으로 물체와의 거리 계산
- 실습에서는 HC-SR04이라는 센서를 활용함.
- 소형 초음파 센서로 많이 활용됨.
- 좌우 약 15도 각도의 범위 내의 2 ~ 400 cm 거리 이내의 물체와의 거리 측정 가능
Ultrasonic sensor 실습
HC-SR04를 이용해 거리 측정을 하여 터미널에 출력하는 실습을 진행해보겠습니다.
실습에 필요한 회로도 입니다.
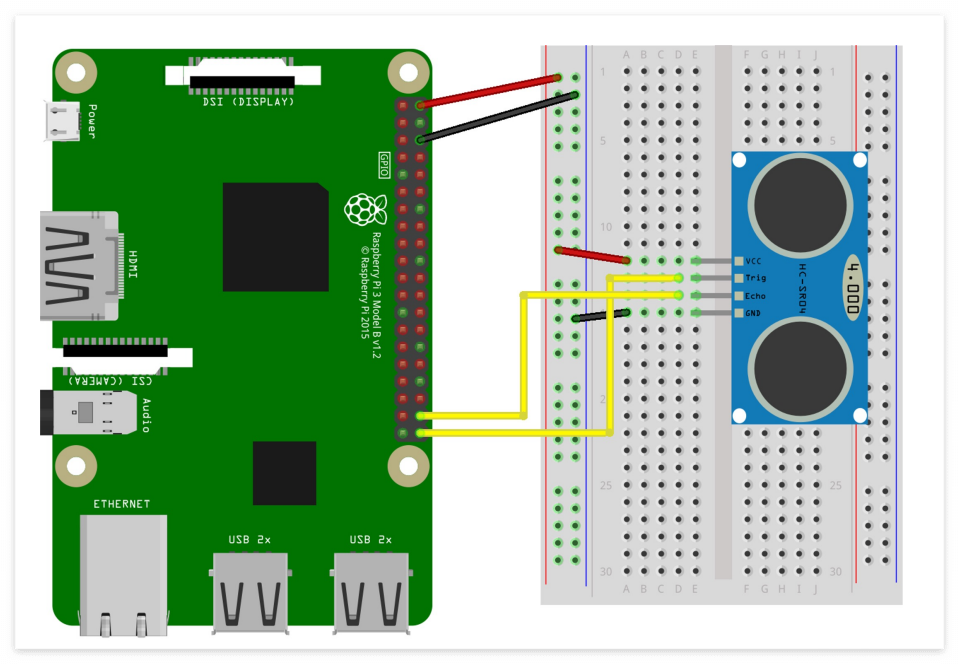
sr04.c 이라는 c파일을 만들어 코드를 작성해보겠습니다.
#include <wiringPi.h>
#include <stdio.h>
#include <stdlib.h>
#include <stdint.h>
#include <unistd.h>
#include <sys/types.h>
int TRIG = 29; //gpio 21 pin 40
int ECHO = 28; //gpio 20 pin 38
static int Echo_Test()
{
unsigned long TX_time = 0;
unsigned long RX_time = 0;
float distance = 0;
unsigned long timeout = 50000000; // 0.5 sec ~ 171 m 50*10^6 us
unsigned long Wait_time=micros();
pinMode(TRIG, OUTPUT); //gpio 21 pin 40 using trigger
pinMode(ECHO, INPUT); //gpio 20 pin 38 using Echo ultra sound
// Ensure trigger is low.
digitalWrite(TRIG, LOW);
delay(50); //mili sec
// Trigger tx sound.
digitalWrite(TRIG, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG, LOW);
// Wait for tx response, or timeout.
while ((digitalRead(ECHO) == LOW && (micros()-Wait_time) < timeout))
{
if(digitalRead(ECHO) == HIGH) break;
}
// Cancel on timeout.
if ((micros()-Wait_time) > timeout)
{
printf("0 Out of range.micros =%d wait-time=%d \n",micros(),Wait_time);
//return 0;
}
TX_time = micros(); //since call wiringPiSetup, the number of microseconds
// Wait for rx sound response, or timeout.
while ((digitalRead(ECHO) == HIGH && (micros()-Wait_time)) < timeout)
{
if(digitalRead(ECHO) == LOW) break;
}
// Cancel on timeout.
if ((micros()-Wait_time) > timeout)
{
printf("1.Out of range.\n");
//return 0;
}
RX_time = micros();
// Calculate rx-tx duration to change distance.
distance = (float) (RX_time - TX_time) * 0.017; //( 340m/2) *100cm/10^6 us
printf("Range %.2f cm.\n", distance);
return 1;
}
int main()
{
printf (" HC-SR04 Ultra-sonic distance measure program \n");
if (wiringPiSetup () == -1)
{
exit(EXIT_FAILURE);
}
if (setuid(getuid()) < 0)
{
perror("Dropping privileges failed.\n");
exit(EXIT_FAILURE);
}
for(;;)
{
Echo_Test();
delay(500); //500ms
//sleep(1);
}
return 0;
}
코드를 작성하였다면 gcc를 이용해 컴파일하고 실행시켜보겠습니다.
pi@raspberrypi:~ $ gcc -o sr04 sr04.c -lwiringPi
pi@raspberrypi:~ $ sudo ./sr04
위의 명령어로 프로그램을 실행하여 거리 센서로 측정된 값을 터미널에 출력 할 수 있습니다.
'Embedded > Raspberry PI' 카테고리의 다른 글
Serial Interfaces (2) | 2023.12.28 |
---|---|
Raspberry PI GPIO 실습(3) (2) | 2023.12.27 |
Raspberry PI GPIO 실습(1) (0) | 2023.12.21 |
Raspberry PI 실습에 사용한 Tool (0) | 2023.12.21 |
Raspberry PI Setup (0) | 2023.12.20 |